How to Stop Your Solidity Code from Guzzling Gas (and ETH!)
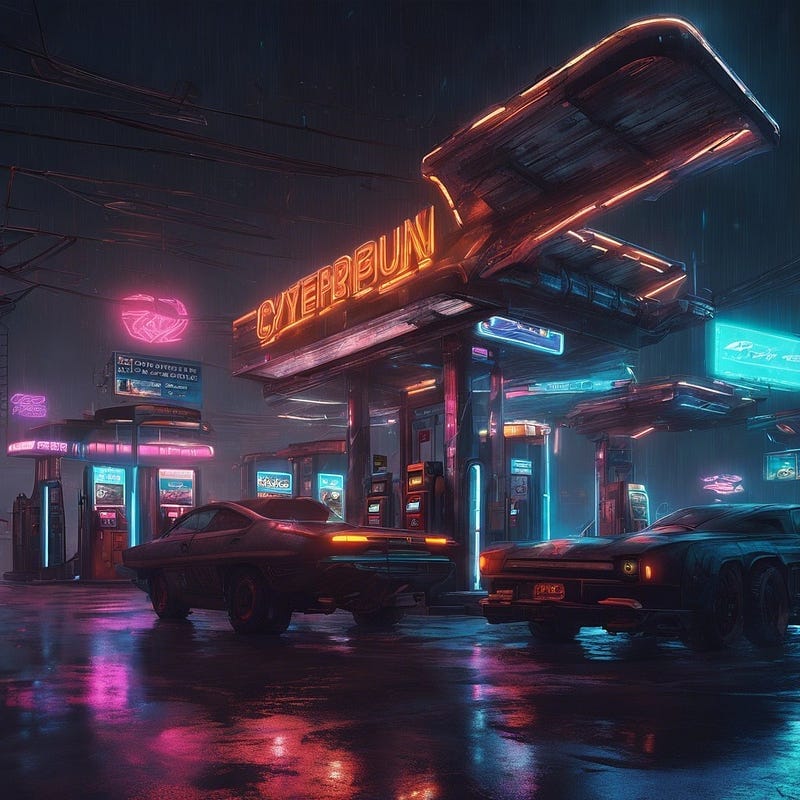
So, you’ve dipped your toes into the wild world of blockchain and built your first smart contract with Solidity. Kudos! But before you pop the champagne and start minting NFTs like there’s no tomorrow, let’s talk about something crucial: gas optimization.
Think of gas as the fuel that powers your smart contract on the Ethereum network. Every line of code, every function call, every little calculation — it all needs gas to run. And just like in the real world, gas is not free. In fact, high gas fees can seriously eat into your profits (and your sanity :) ).
That’s where gas optimization comes in. It’s the art of writing smart contracts that are lean and efficient, so they consume less gas and save you precious ETH or whatever you pay for it.
Why Should You Care About Gas Optimization?
Imagine this: you’ve created an awesome decentralized app (DApp) that everyone’s raving about. But then, users start complaining about exorbitant transaction fees. Suddenly, your dream DApp becomes too expensive to use, and people lose interest. Ouch.
Optimizing your Solidity code for gas efficiency isn’t just about saving a few bucks… it’s about:
- Accessibility: Lower gas fees make your dApp more accessible to a wider audience.
- Sustainability: Less gas consumption means a smaller carbon footprint for your project.
- User Experience: Nobody likes waiting for slow and expensive transactions. Gas optimization makes your dApp snappier and more enjoyable to use.
Solidity Gas Optimization: Let’s Get Technical (But Not Too Technical)
Don’t worry, this isn’t going to be a dry and boring lecture on assembly code. We’ll stick to practical tips that even beginner Solidity developers can understand and implement.
1. Data Structures: Choose Wisely, My Friend
- Arrays: Avoid unnecessary array resizing. Pre-allocate memory if you know the size beforehand. Remember, pushing data into a dynamically sized array is like trying to fit an elephant into a Mini Cooper — it’s gonna cost you!
- Mappings: Use mappings whenever possible. They’re like hash tables, offering super-fast lookups and saving you gas compared to iterating through arrays to find what you need.
2. Loops: The Bane of Gas-Guzzlers
- Minimize Loop Iterations: Keep those loops tight! The fewer times you have to loop, the less gas you’ll burn.
- Don’t Loop What You Can Calculate: If you can calculate a value directly instead of looping to find it, do it! Your gas tank will thank you.
3. Functions: Short, Sweet, and Gas-Efficient
- Internal vs. External: Use
internal
functions whenever possible. They're only callable from within the contract and are cheaper thanexternal
functions. - Pure vs. View: If a function doesn’t modify the blockchain state, declare it as
view
(for reading data) orpure
(for calculations only). These functions don't consume any gas when called externally.
4. Variables: Pack ’Em In Like Sardines
- Variable Packing: Solidity allows you to pack multiple variables into a single storage slot. This might sound like some black magic, but it’s a legit way to save gas on storage.
- Use Unsigned Integers: When you know a value will always be positive, use an
uint
instead of anint
. Unsigned integers take up less space and are cheaper to work with.
5. Libraries: Don’t Reinvent the Wheel
- Leverage Existing Libraries: Chances are, someone has already solved a problem you’re facing. Use well-tested and optimized libraries to save time and gas.
FAQs: Your Burning Gas Optimization Questions, Answered
1. What is the most important thing to remember about gas optimization?
Keep it simple! The more complex your code, the more gas it will consume. Write clean, concise, and readable code, and you’ll be on the right track.
2. How can I estimate the gas cost of my Solidity code?
Remix, the popular Solidity IDE, has a built-in gas estimator that can give you a rough idea of how much gas your code will consume. Hardhat has special plugin called hardhat-gas-reporter to measure gas consumption during tests. In foundry you can simply run tests with gas tracker flag like this: forge test --gas-report
3. Are there any tools that can help me optimize my Solidity code?
Yes! There are several tools available, such as:
- Solhint: A linter that helps you identify potential code style and security issues, some of which can impact gas efficiency. [site]
- Mythril: A security analysis tool that can also detect gas optimization opportunities. [git]
4. Is gas optimization a one-time thing?
Not really. As your project evolves and you add new features, it’s crucial to revisit your code and optimize for gas efficiency regularly.
5. I’m a beginner. Do I really need to worry about gas optimization right now?
While it’s essential to understand the basics of gas optimization from the get-go, don’t get bogged down by it when you’re just starting. Focus on writing clean and functional code first, and then gradually incorporate optimization techniques as you gain more experience.
Gas Optimization: Your Key to a Smoother Blockchain Journey
Optimizing your Solidity code for gas efficiency is an ongoing process, but its a worthwhile investment that will pay dividends in the long run. By following these tips and staying up-to-date with the latest best practices, you can ensure that your DApps are accessible, sustainable, and a joy to use.